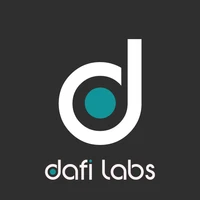
ai vs human image detection
DafilabIntroduction
The AI vs Human Image Detection model is designed to distinguish between AI-generated and human-created images. Built using the EfficientNet-B4 architecture and the PyTorch framework, it achieves high accuracy in classifying images from a dataset evenly split between AI and human sources.
Architecture
- Model Architecture: EfficientNet-B4
- Framework: PyTorch
- Base Model:
google/efficientnet-b4
- Library:
timm
Training
The model was trained on approximately 100,000 AI-generated images and 100,000 human-created images. It demonstrates impressive performance with the following evaluation metrics:
- Training Accuracy: 99.75%
- Validation Accuracy: 98.59%
- Training Loss: 0.0072
- Validation Loss: 0.0553
Guide: Running Locally
-
Install Dependencies:
pip install torch torchvision timm huggingface_hub pillow
-
Download Model: Use the Hugging Face Hub to download the model file.
from huggingface_hub import hf_hub_download MODEL_PATH = hf_hub_download(repo_id="Dafilab/ai-vs-human-image-detection", filename="model_epoch_8_acc_0.9859.pth")
-
Load and Prepare the Model: Use PyTorch and Timm to load the model and prepare it for inference. Ensure you have a GPU for faster computation if available.
import torch from timm import create_model DEVICE = torch.device("cuda" if torch.cuda.is_available() else "cpu") model = create_model('efficientnet_b4', pretrained=False, num_classes=2) model.load_state_dict(torch.load(MODEL_PATH, map_location=DEVICE)) model.to(DEVICE).eval()
-
Run Predictions: Define a prediction function to classify new images.
from torchvision import transforms from PIL import Image def predict_image(image_path): img = Image.open(image_path).convert("RGB") img = transform(img).unsqueeze(0).to(DEVICE) with torch.no_grad(): logits = model(img) probs = torch.nn.functional.softmax(logits, dim=1) predicted_class = torch.argmax(probs, dim=1).item() confidence = probs[0, predicted_class].item() return LABEL_MAPPING[predicted_class], confidence # Example usage image_path = "path/to/image.jpg" label, confidence = predict_image(image_path) print(f"Label: {label}, Confidence: {confidence:.2f}")
-
Suggested Cloud GPUs: Consider using cloud services such as AWS EC2, Google Cloud Platform, or Azure for GPU access to improve model inference speed.
License
This project is licensed under the Apache License 2.0.