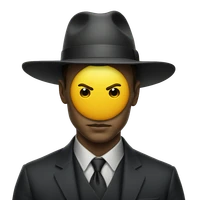
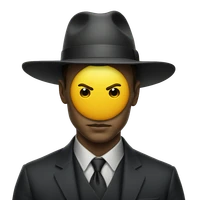
Other
Text Generation
Text To Image
Text Classification
Fill Mask
Text2text Generation
Automatic Speech Recognition
Image Text To Text
Sentence Similarity
Token Classification
Feature Extraction
Translation
Image Classification
Text To Speech
Question Answering
Image To Image
Summarization
Object Detection
Image Segmentation
Image To Text
Text To Video
Audio Classification
Zero Shot Image Classification
Image Feature Extraction
Zero Shot Classification
Audio To Audio
Visual Question Answering
Reinforcement Learning
Image To Video
Text To Audio
Video Classification
Unconditional Image Generation
Time Series Forecasting
Video Text To Text
Any To Any
Audio Text To Text
Depth Estimation
Text To 3d
Image To 3d
Robotics
Mask Generation
Table Question Answering
Voice Activity Detection
Document Question Answering
Keypoint Detection
Zero Shot Object Detection
Tabular Classification
Graph Ml
Tabular Regression
More Related APIs in Video Text To Text
L La V A Video 7 B Qwen2
Long V U_ Llama3_2_1 B
Intern Video2_5_ Chat_8 B
Video Chat Flash Qwen2_5 2 B_res448
L La V A Ne X T Video 7 B hf
Video Chat Flash Qwen2 7 B_res448
cogvlm2 llama3 caption
Video Chat Flash Qwen2 7 B_res224
Space Time G P T
Newsletter
Get updates on new LLM models, and other cool AI stuff.