Other
Text Generation
Text To Image
Text Classification
Fill Mask
Text2text Generation
Automatic Speech Recognition
Image Text To Text
Sentence Similarity
Token Classification
Feature Extraction
Translation
Image Classification
Text To Speech
Question Answering
Image To Image
Summarization
Object Detection
Image Segmentation
Image To Text
Text To Video
Audio Classification
Zero Shot Image Classification
Image Feature Extraction
Zero Shot Classification
Audio To Audio
Visual Question Answering
Reinforcement Learning
Image To Video
Text To Audio
Video Classification
Unconditional Image Generation
Time Series Forecasting
Video Text To Text
Any To Any
Audio Text To Text
Depth Estimation
Text To 3d
Image To 3d
Robotics
Mask Generation
Table Question Answering
Voice Activity Detection
Document Question Answering
Keypoint Detection
Zero Shot Object Detection
Tabular Classification
Graph Ml
Tabular Regression
More Related APIs in Text Generation
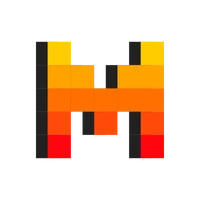
Mistral Small 24 B Instruct 2501
Text Generation
4 days ago
24K
614
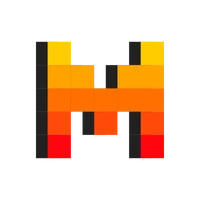
Mistral Small 24 B Base 2501
Text Generation
7 days ago
3.7K
195
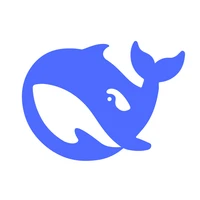
Deep Seek R1 Distill Qwen 32 B
Text Generation
5 days ago
382.1K
890
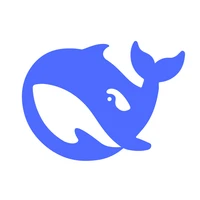
Deep Seek R1 Distill Qwen 1.5 B
Text Generation
5 days ago
423.3K
676
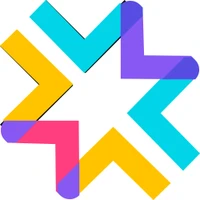
Yu E s1 7 B anneal en cot
Text Generation
7 days ago
22.7K
344
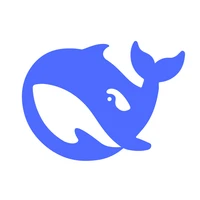
Deep Seek R1 Zero
Text Generation
5 days ago
24.8K
716
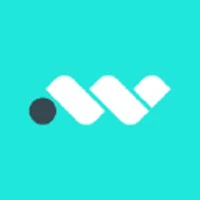
Velvet 14 B
Text Generation
6 days ago
2.3K
107
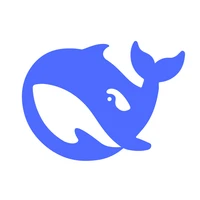
Deep Seek R1 Distill Llama 70 B
Text Generation
5 days ago
170.7K
466
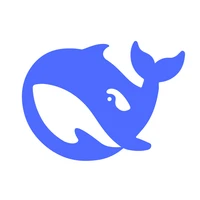
Deep Seek R1 Distill Llama 8 B
Text Generation
5 days ago
285.9K
428
Newsletter
Get updates on new LLM models, and other cool AI stuff.Made by Mohd Danish & Data from Hugging Face