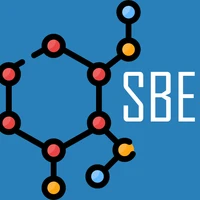
paraphrase albert small v2
sentence-transformersIntroduction
The paraphrase-albert-small-v2
model is part of the Sentence-Transformers library, designed for mapping sentences and paragraphs into a 768-dimensional dense vector space. This model is suitable for tasks such as clustering and semantic search.
Architecture
The model architecture includes:
- A Transformer component utilizing
AlbertModel
with a maximum sequence length of 100. - A Pooling layer that applies mean pooling to word embeddings, which results in a 768-dimensional sentence vector.
Training
The model was trained by the Sentence-Transformers team, as mentioned in their publication "Sentence-BERT: Sentence Embeddings using Siamese BERT-Networks".
Guide: Running Locally
To use the model, follow these steps:
-
Install Sentence-Transformers:
pip install -U sentence-transformers
-
Use the model with Sentence-Transformers:
from sentence_transformers import SentenceTransformer sentences = ["This is an example sentence", "Each sentence is converted"] model = SentenceTransformer('sentence-transformers/paraphrase-albert-small-v2') embeddings = model.encode(sentences) print(embeddings)
-
Use the model with Hugging Face Transformers:
from transformers import AutoTokenizer, AutoModel import torch def mean_pooling(model_output, attention_mask): token_embeddings = model_output[0] input_mask_expanded = attention_mask.unsqueeze(-1).expand(token_embeddings.size()).float() return torch.sum(token_embeddings * input_mask_expanded, 1) / torch.clamp(input_mask_expanded.sum(1), min=1e-9) sentences = ['This is an example sentence', 'Each sentence is converted'] tokenizer = AutoTokenizer.from_pretrained('sentence-transformers/paraphrase-albert-small-v2') model = AutoModel.from_pretrained('sentence-transformers/paraphrase-albert-small-v2') encoded_input = tokenizer(sentences, padding=True, truncation=True, return_tensors='pt') with torch.no_grad(): model_output = model(**encoded_input) sentence_embeddings = mean_pooling(model_output, encoded_input['attention_mask']) print("Sentence embeddings:") print(sentence_embeddings)
For enhanced performance, consider utilizing cloud GPUs such as those offered by AWS, Google Cloud, or Azure.
License
The model is licensed under the Apache-2.0 license, allowing for both personal and commercial use.