Other
Text Generation
Text To Image
Text Classification
Fill Mask
Text2text Generation
Automatic Speech Recognition
Image Text To Text
Sentence Similarity
Token Classification
Feature Extraction
Translation
Image Classification
Text To Speech
Question Answering
Image To Image
Summarization
Object Detection
Image Segmentation
Image To Text
Text To Video
Audio Classification
Zero Shot Image Classification
Image Feature Extraction
Zero Shot Classification
Audio To Audio
Visual Question Answering
Reinforcement Learning
Image To Video
Text To Audio
Video Classification
Unconditional Image Generation
Time Series Forecasting
Video Text To Text
Any To Any
Audio Text To Text
Depth Estimation
Text To 3d
Image To 3d
Robotics
Mask Generation
Table Question Answering
Voice Activity Detection
Document Question Answering
Keypoint Detection
Zero Shot Object Detection
Tabular Classification
Graph Ml
Tabular Regression
More Related APIs in Automatic Speech Recognition
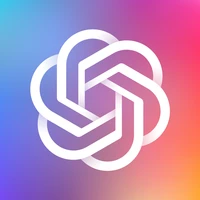
whisper large v3 turbo
Automatic Speech Recognition
4 months ago
3.3M
1.9K
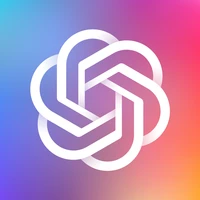
whisper large v3
Automatic Speech Recognition
6 months ago
4.8M
4K
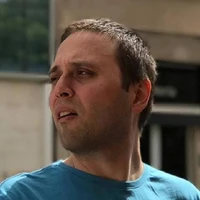
whisper.cpp
Automatic Speech Recognition
3 months ago
0
881
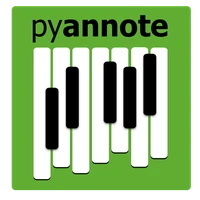
speaker diarization 3.1
Automatic Speech Recognition
9 months ago
10.6M
671
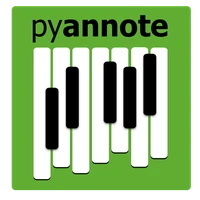
speaker diarization
Automatic Speech Recognition
9 months ago
6.4M
937
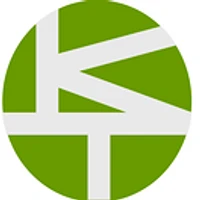
kotoba whisper v2.0
Automatic Speech Recognition
3 months ago
6.2K
47
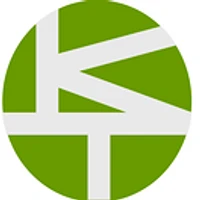
kotoba whisper v2.2
Automatic Speech Recognition
3 months ago
6.5K
29
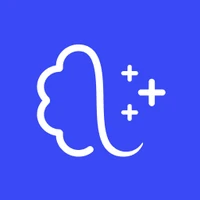
Crisper Whisper
Automatic Speech Recognition
2 months ago
43.7K
224
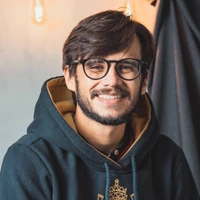
wav2vec2 large xlsr 53 english
Automatic Speech Recognition
2 years ago
20.6M
456
Newsletter
Get updates on new LLM models, and other cool AI stuff.Made by Mohd Danish & Data from Hugging Face