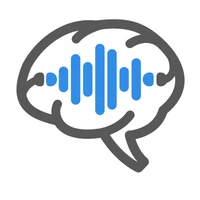
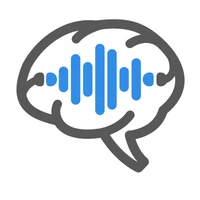
Other
Text Generation
Text To Image
Text Classification
Fill Mask
Text2text Generation
Automatic Speech Recognition
Image Text To Text
Sentence Similarity
Token Classification
Feature Extraction
Translation
Image Classification
Text To Speech
Question Answering
Image To Image
Summarization
Object Detection
Image Segmentation
Image To Text
Text To Video
Audio Classification
Zero Shot Image Classification
Image Feature Extraction
Zero Shot Classification
Audio To Audio
Visual Question Answering
Reinforcement Learning
Image To Video
Text To Audio
Video Classification
Unconditional Image Generation
Time Series Forecasting
Video Text To Text
Any To Any
Audio Text To Text
Depth Estimation
Text To 3d
Image To 3d
Robotics
Mask Generation
Table Question Answering
Voice Activity Detection
Document Question Answering
Keypoint Detection
Zero Shot Object Detection
Tabular Classification
Graph Ml
Tabular Regression
More Related APIs in Text To Speech
Kokoro 82 M
X T T S v2
Llasa 3 B
Llasa 8 B
F5 T T S
fish speech 1.5
Tsukasa_ Speech
Cosy Voice2 0.5 B
F5 T T S pt br
Newsletter
Get updates on new LLM models, and other cool AI stuff.